[Rust] — Rust’s concepts intuitively explained — Part 1(Visualized)
Rust programming language takes a unique approach to address common issues found in other languages, with a robust type system that…
![[Rust] — Rust’s concepts intuitively explained — Part 1(Visualized)](/content/images/size/w1200/2025/03/0-7hrkrmkf2wbtiqvb.png)
Rust programming language takes a unique approach to address common issues found in other languages, with a robust type system that promotes explicitness and clarity. However, modern problems require modern abstractions. In this post, I aim to provide a simplified and intuitive explanation of Rust concepts and data types, making it easier for you to navigate and understand this powerful language.
Null Object and the null object exception
Other languages
When passing an object to a function, there’s no guarantee that the function won’t assign the parameter to NULL. This can lead to unexpected issues when attempting to use the object later, resulting in possible NULL references and exceptions.
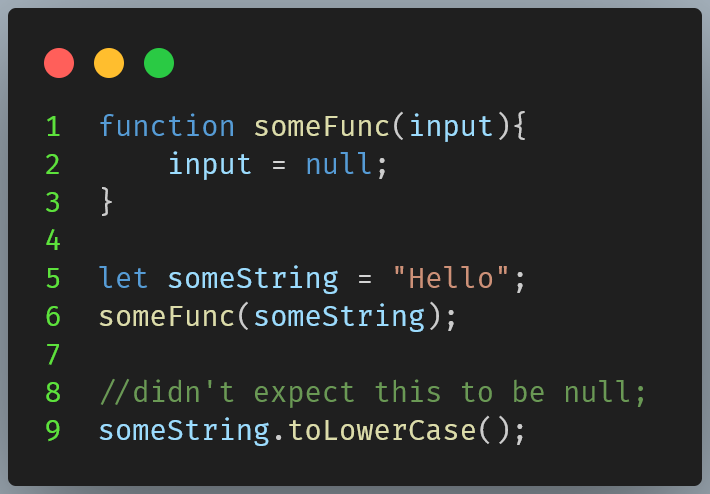
Imagine lending a toy to your friend using a post-office service. In order to send the toy, you carefully place it inside a box and ship it over. However, when your friend returns the toy, you excitedly open the box, only to find it completely empty.
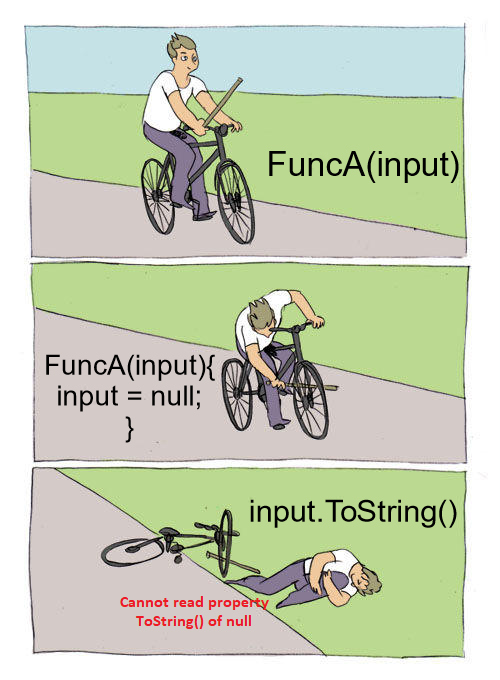
Dealing with null parameters in our functions can be quite tedious. Unfortunately, if we forget to perform the null check, our program can end up exploding.

Imagine this scenario from your friend’s perspective: when they send you a box through the post office, they expect that when you open it, you’ll find the toy inside. However, imagine their disappointment when you open the box and discover that it’s empty.
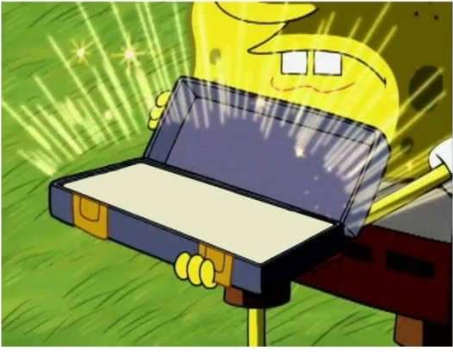
The Rust way
Rust doesn’t have NULL.
Without NULL value, the function cannot set it’s param to NULL.
The post office has implemented a new policy where they only accept non-empty boxes. As a result, when someone returns the toy to you, you can rest assured that you will never receive an empty box.
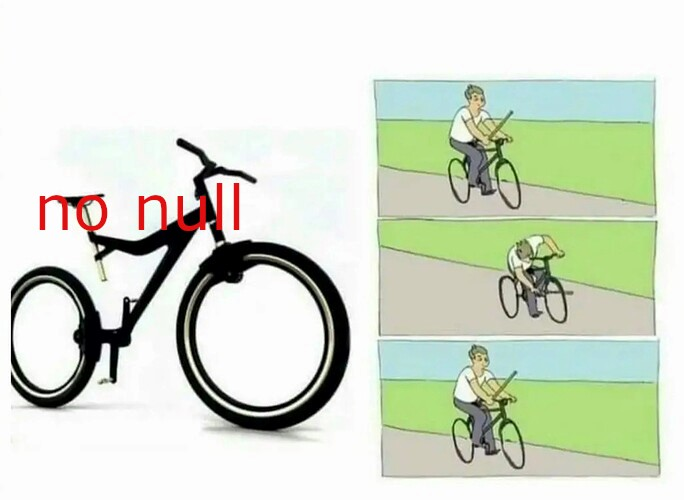
Nor your friend receive empty box when he borrows something.
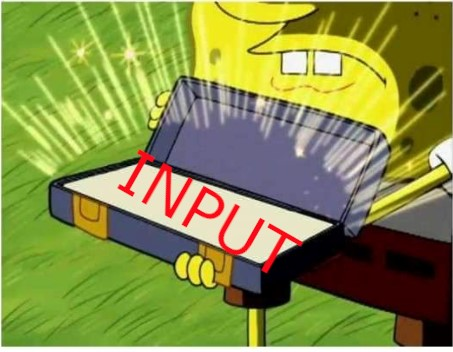
Ownership
Rust has ownership model with 3 rules:
- Each value in Rust has an owner (owner is a function)
- There can only be one owner at a time.
- When the owner goes out of scope, the value will be dropped.
This time i will give you another analogy.
You can think of a Car. It can only be legally owned by one person at the time, he can let others borrow it, then others can let someone borrow it again or ask him to change color or its part but can never sell it to other person. Because they don’t have the legal ownership over that car.
Back to the NULL problem earlier, with ownership model Rust prevents the function from reassign it’s parameter unless it requires ownership over that parameter.
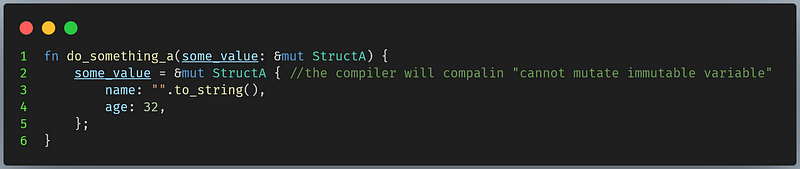
You cannot swap a car to another car. It’s not identical to the original car. More important, you do not own it, if you own it you can swap it to whatever you want.
If we give ownership to the function, we won’t be able to use that variable after that function call. Hence, there’s no chance we get unexpected behavior.
This also works really well when you do multi-threaded programming. thread::spawn
requires ownership over it’s closure (there are special cases, we will get to them later). Hence, if the thread lives longer than it’s parent, our code won’t fail because the spawned thread owns everything in its scope, all of them will be destroyed when the thread ends.
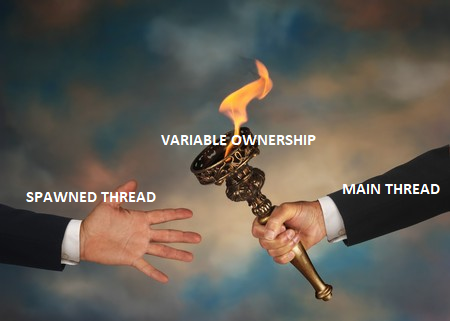
Think of this as the car cannot be driven by multiple person at multiple location at the same time.
Lifetime
Lifetime means how long a value lives till it gets destroyed.
I will give you a real life analogy using Rust Code
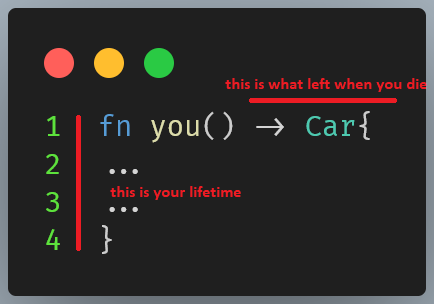
Here’s your lifetime, you buy a car, do whatever you want with it and transfer the ownership of the car when you die. This code compile 👌
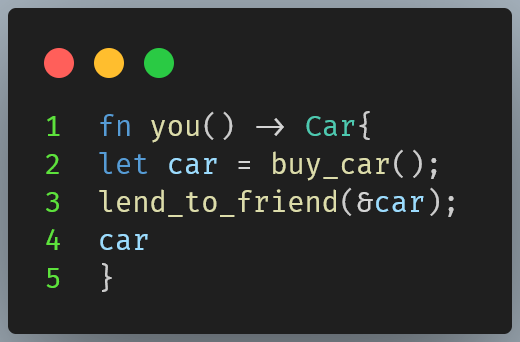
When you die, you cannot let someone borrow it because it just doesn’t make sense. If someone borrow it, they borrow from who? you are no longer alive.
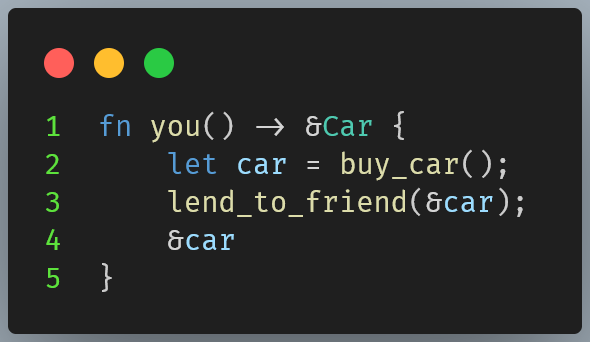
But when you die, you return something you borrowed, it makes totally sense
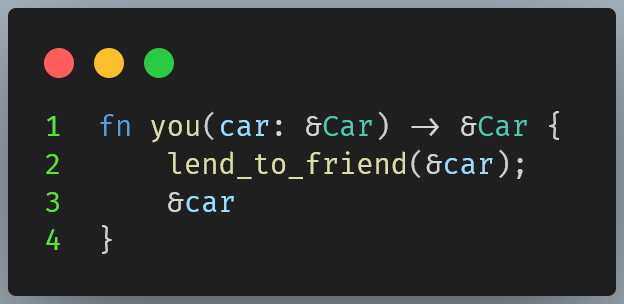
Conclusion
I hope this post helps you grasp the concepts Rust provides.
I planned to make this a long post but it would be overwhelming putting everything inside a blog post.
If you like the post, give me a clap and follow me for new posts in the future.