[Rust] The Closure I needed
At first glance, the Fn family in Rust may appear daunting, but once you grasp the purpose and rationale behind their existence, you’ll…
![[Rust] The Closure I needed](/content/images/size/w1200/2025/03/1-3j_ok2rwv2o35k171s4ala.png)
At first glance, the Fn family in Rust may appear daunting, but once you grasp the purpose and rationale behind their existence, you’ll appreciate their distinctive qualities.
Fn and closure in other languages
Function pointer (delegate)
Function pointer or delegate in different programming languages can go by various names, including callback, delegate, anonymous function, or any other term.
Here’s an example written in C#
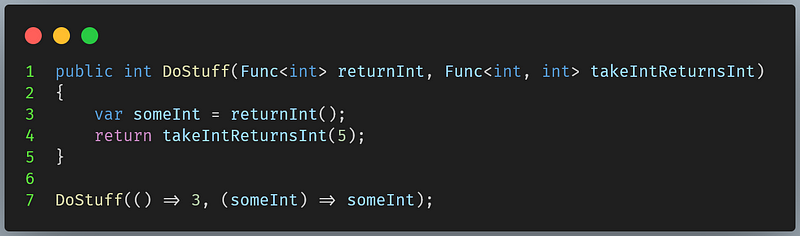
And an example in Typescript

Closure
A closure, in other languages are same as delegate,except that they can access variables outside of its own scope. This means that a closure captures its surrounding environment, enabling it to retain and utilize those variables within its execution context.
Here’s the above c# version example but the delegate has access to variable outside of it’s scope
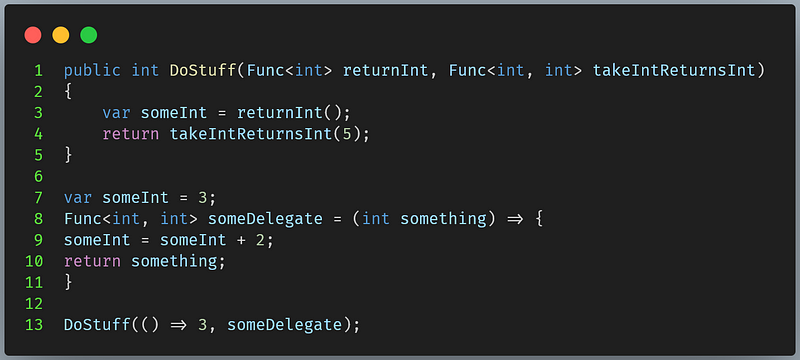
In the preceding example, someDelegate
access to someInt
, everytime DoStuff
invokes the takeIntReturnsInt
, the someInt is increased by 2
. Magic!!!
Example in Typescript
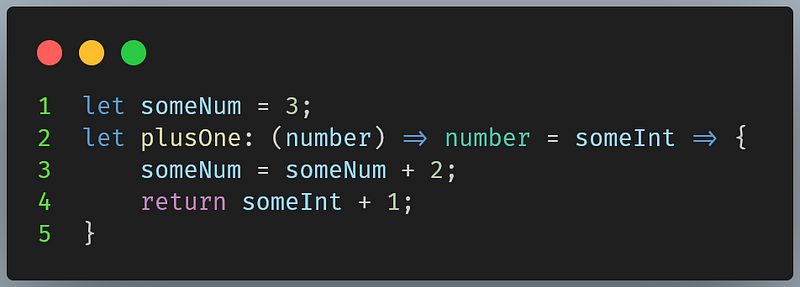
This approach is incredibly convenient, user-friendly, and holds great potential in this context. However, it is important to remember that great power comes with great responsibility. We must take into account situations where the closure attempts to access a disposed resource. Additionally, in multi-threaded scenarios, managing variable mutations can become even more complex.
Fn in Rust
Rust, with its ownership model, takes a distinct approach. The traits Fn
, FnMut
, and FnOnce
govern how closures utilize captured variables, differing primarily in their manner of usage.
FnOnce
The FnOnce
trait represents a closure that takes ownership of the captured variables. It’s aptly named Once
because it consumes them.
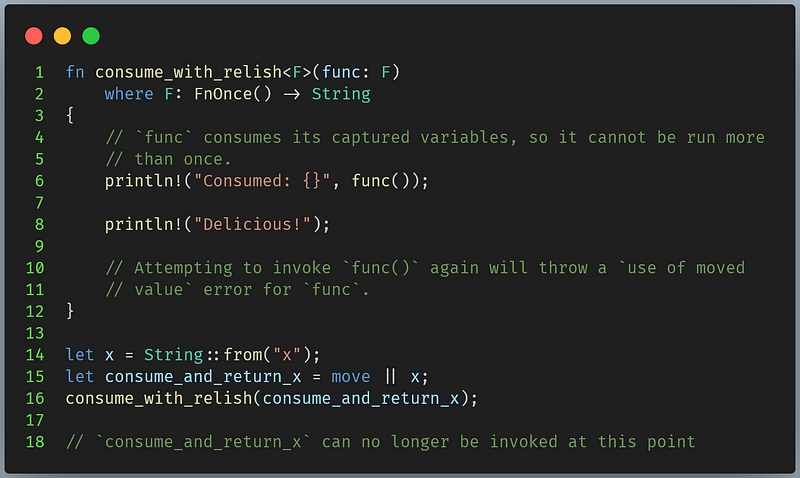
The move
keyword is used when using FnOnce
to indicate taking ownership of the variable x
.
FnMut
The `FnMut` trait represents a closure that has a mutable reference to the captured variables. In the example below, we demonstrate how to mutate the captured variable within the closure.
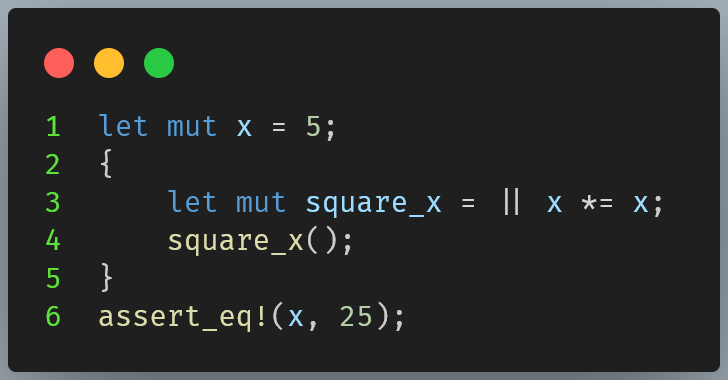
Fn
Fn
trait represent a closure which has immutably reference to the captured variables but doesn’t change anything. This should looks familiar with the typescript version of closure.
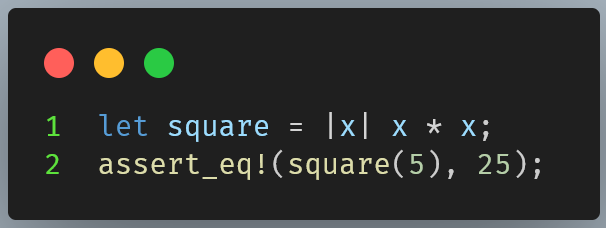
fn (Function Pointer)
fn
is a function pointer, do not confuse with the Fn
closure. One important thing is that Fn-family is trait
and fn is a type
.
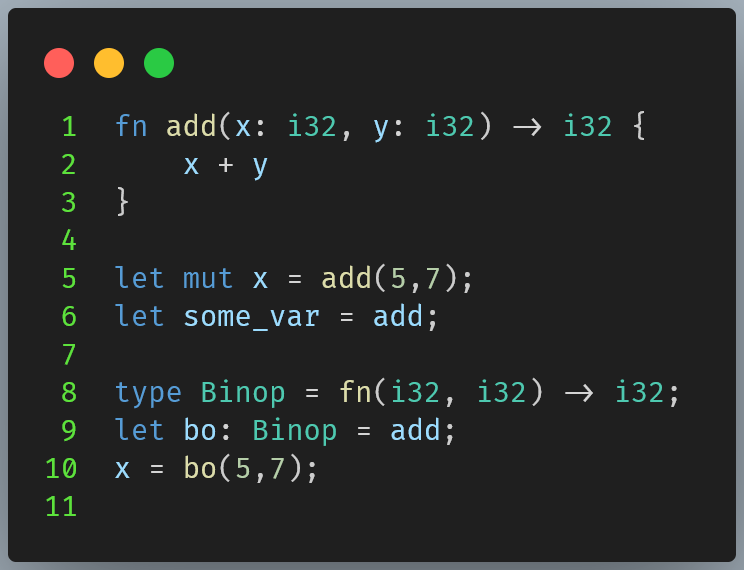
In the previous example, we assigned the add
function to the variable some_var
. We can also create an alias type with the same parameter signature and assign the add
function to a variable of that new type
Conclusion
The Fn-family might seem confusing initially, but as you become more accustomed to using them, you’ll appreciate the explicitness and differentiation that Rust provides.
If you enjoyed this post, don’t forget to give it a clap and follow me for updates on future content