The For Loop vs Foreach Loop: The Ultimate Showdown
In C#, there are two main types of loops: the for loop and the foreach loop. The for loop is a general-purpose loop that can be used to…
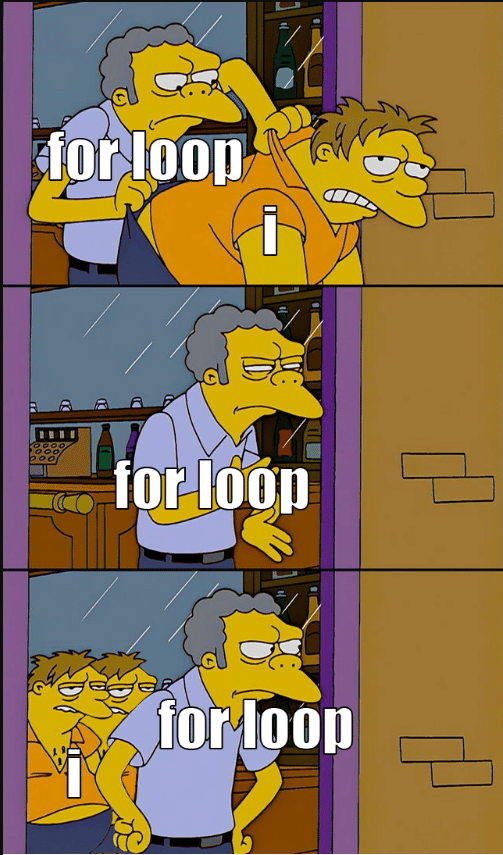
In C#, there are two main types of loops: the for
loop and the foreach
loop. The for
loop is a general-purpose loop that can be used to iterate over any sequence of data, while the foreach
loop is a special-purpose loop that can only be used to iterate over collections.
In this article, we will discuss the differences between the for
and foreach
loops in C#. We will also provide some examples of how to use each type of loop.
The for loop
The for
loop is a general-purpose loop that can be used to iterate over any sequence of data. It has the following syntax:
for (initialization; condition; increment) {
statements;
}
The initialization
expression is executed once, before the loop begins. The condition
expression is evaluated before each iteration of the loop. If the condition expression evaluates to true
, the loop body is executed. Otherwise, the loop terminates. The increment
expression is executed after each iteration of the loop.
The initialization
expression can be any valid C# expression. It is often used to declare and initialize a loop counter variable. The condition
expression must be a Boolean expression. If the condition expression evaluates to false
when the loop first starts, the loop body will not be executed even once.
The increment
expression is also any valid C# expression. It is often used to increment or decrement the loop counter variable.
Here is an example of a for
loop that prints the numbers from 0 to 9:
for (int i = 0; i < 10; i++) {
Console.WriteLine(i);
}
We can also increase i by a value of your choosing, such as 10.
for (int i = 0; i < 100; i += 10) {
Console.WriteLine(i);
}
Using for loop this way, we can arbitrarily access the array using index.
Indexer
Indexers allow instances of a class or struct to be indexed just like arrays. The indexed value can be set or retrieved without explicitly specifying a type or instance member. Indexers resemble properties except that their accessors take parameters.
using System;
class SampleCollection<T>
{
// Declare an array to store the data elements.
private T[] arr = new T[100];
// Define the indexer to allow client code to use [] notation.
public T this[int i]
{
get { return arr[i]; }
set { arr[i] = value; }
}
}
class Program
{
static void Main()
{
var stringCollection = new SampleCollection<string>();
stringCollection[0] = "Hello, World";
Console.WriteLine(stringCollection[0]);
}
}
// The example displays the following output:
// Hello, World.
The foreach loop
The foreach
loop is a special-purpose loop that can only be used to iterate over collections. It has the following syntax
foreach (element in collection) {
statements;
}
The element
variable is a temporary variable that is used to hold each element in the collection as it is iterated over. The collection
variable must be a reference to a collection that implements the IEnumerable
interface.
The statements
block is executed for each element in the collection. The statements
block can contain any valid C# statements.
The foreach
loop can only be used to iterate over collections that implement the IEnumerable
interface
Reassign value in foreach loop
We cannot reassign the value of the element variable in a foreach
loop in C#. The element variable is a temporary variable that is used to hold each element in the collection as it is iterated over. It is not a reference to the actual element in the collection. If we were to compile this code, it would not compile.
var names = new List<string> { "Harry", "Ron", "Hermione" };
foreach (var name in names)
{
//cannot assign to 'name' because it is a 'foreach iteration variable'
name = "Voldermort";
}
Add or remove element in Foreach loop
We cannot add or remove values in a collection using a foreach
loop in C#. The foreach
loop is a read-only loop, which means that it can only iterate over the collection and cannot modify it.
List<int> list = new List<int>{
1
};
foreach (var num in list)
{
list.Add(num + 1);
Console.WriteLine(num);
}
The code would throw System.InvalidOperationException: Collection was modified; enumeration operation may not execute
.
The real power of Foreach
The real power of foreach
comes when you need to iterate over a collection that is lazy or has an unfixed length. For example, the Fibonacci sequence or the prime number sequence.
Lazy execution means that the collection is not created until it is needed. This can be useful for large or infinite collections.
Unfixed length means that the number of items in the collection can change at any time. This can be useful for collections that are constantly being updated, such as a list of users who are online.
In these cases, a foreach
loop is a better choice than a for loop because it does not require the entire collection to be created or have a fixed length. This can save memory and processing time.
IEnumerable<int> GetCollection()
{
int i = 0;
while (true)
{
yield return i;
i += 1;
}
}
var items = GetCollection();
foreach (var item in items)
{
Console.WriteLine(item);
if (item == 100)
{
break;
}
}
We can’t do that with a for
loop because IEnumerable
doesn’t have an index.
for(int i = 0; i < ??; i++){
}
Conclusion
For
loop is more flexible when it comes to accessing and assigning values inside the loop because you can do whatever you want with the index. But foreach
loop is easier when you just want to iterate over the collection sequentially, or when you don’t know how many items are in the collection.
Here’s a friendly reminder “Use the right tool for the job.”